Hi Rebirth, this is being started as an amatuer project. I really don't know what I'm doing. but I've started anyways.
What am I doing? I have copied some of the broken sourcecode for the old Suckerpunch's hero planner, which was an early webpage version of what would eventually inspire Mids. The goal is to modify, fix and update that code to the current database version of rebirth mids (and eventually others) to produce a page where you can plan your hero/villain builds in any webbrowser without any login. so if you save the page you could even use it offline.
Long story short, there has been some interest in a hero/villain planner that can be used on all devices. The Mids team has plans for such a thing (though not actually a webpage version), but they have expressed it's not going to be in the near future as they are focusing on current mids dev which is an extensive task.
Source: Suckerpunch's sourcecode can be found on
archive.org from the old cohtitan page for it. the software at that time required you to be logged in to cohtitan to use it, as you cannot validly login to cohtitan via
archive.org and as even if you did so the titan staff have long since taken the necessary files to support suckerpunch's offline; the code is effectively broken. but you can find it here:
view-source:
https://web.archive.org/web/20110824142004/http://planner.cohtitan.com/planner
and the broken planner looks like this:
https://web.archive.org/web/20110824142004/http://planner.cohtitan.com/planner
Progress:
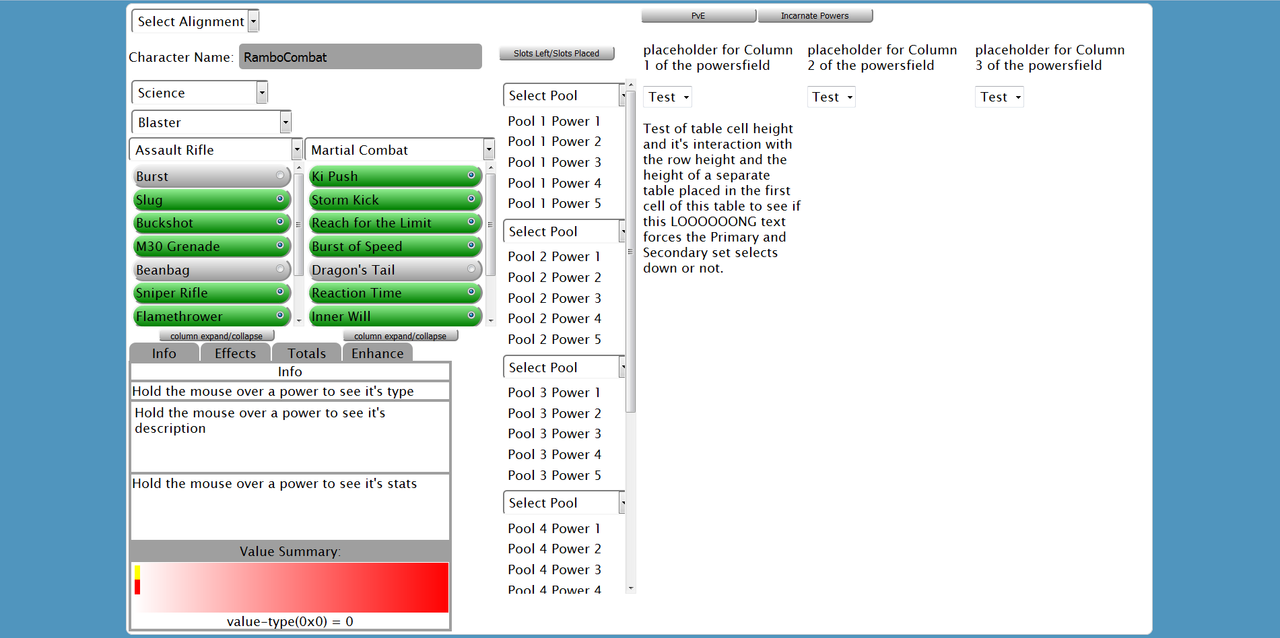
New hero crest image for the background. spent all day making it. I added it tot he background in the doc. This image will end up in a zip of images later as more images become necessary so as to save attachment space.
[attachment id=0 msg=4490]
I have also stripped off the log-in arguments.
Right now: KEYBOARD REPLACED. It's been a long time. I got back into it today and started inputting the secondary power selections. Blaster's secondaries are all selectable (but still no data and no anything else). I'm not going to try to upload the script today, I'll wait till it's done. I'll upload a new image of the state of things so far tomorrow or something.
Also I'm thinking up a better method to do the tabs in the power info/totals table. I'm hesitant on using position:absolute and the z axis to control which tab's content is visible, instead I'm literally building the table from code on tab click. the downside for that is I'll need somewhere to store dynamic power info for the table, based on which power last had the mouse over it or is locked. I'm thinking a hidden object, with the data printed on it. could make a button to make it visible but it'll be multiple tabs worth of info so, a lot of text.
Next: Do the whole process again for power pools and Ancillary/Patron pools. And I plan to rework the power info table and tabs to be on different Z locations with backgrounds on the table cells so the can literally work as real tabs.
After that: Create Power Info Dictionaries and a power info table to display the information from the dictionary on button select or mouse-over.
Even Further: The Chosen Powers Field
Future concerns: the mids team has noted that Javascript's floating point numbers might not be compatible with City of heroes numbers. I have talked with them about this and determined that javascript with floats set to a precision of 4 to 6 decimal places might be able to equate it to CoH's floats.
Because of that there is a chance that even if I can make a visibly working planner in a webpage, the numbers wont be right and it may be worthless.

but whatever lets try anyways.. maybe thats why suckerpunch's was taken down, though I seem to remember it was taken down because the guy that made it did not want to maintain it.
This is an open project, if someone knows more than me and wants to spearhead it, by all means please do so, I look forwards to your leadership lol. I'd share my test page, but I'm afraid people would visit my main page. my site is a joke and so I don't feel comfortable sharing it. a site written by a madman for the purpose of html testing and trolllery.. it was supposed to be a portfolio page.. yeah.. troll+freewebpage to do whatever with = webpage poop. If my progress gets closer to something that looks usable, I will clean the poop off my site and share it.
Meanwhile I'll share the source code so far:
CSS buildmaker.css an incomplete cropping of suckerpunch's planner.css with just the bits I need to make what I have work (and some others I have yet to know if I need them)
body, div, span, ul, li, select { margin: 0; padding: 0.2vw; font-family: "Lucida Sans Unicode", "Lucida Grande", Verdana, Arial, sans-serif; font-size: 1vw; }
body {
background: #5095BE
}
#CoXBuildMaker_body { width: 75%; margin: 10px auto 0; position: relative; background: white; border: 1px solid #CCC; border-radius: 10px; -moz-border-radius: 10px; -webkit-border-radius: 10px; overflow: hidden;}
select option[value="Hero"]{
margin: 40px;
background: #5095BE;
color:#fff;
text-shadow: 0 1px 0 rgba(0, 0, 0, 0.4);
font-size: 1vw;
}
select option[value="Villain"]{
margin: 40px;
background: #990000;
color:#fff;
text-shadow: 0 1px 0 rgba(0, 0, 0, 0.4);
font-size: 1vw;
}
div.powerSelection {
border-right: 0.2vw solid #909090;
border-bottom: 0.3vh solid #909090;
border-top: 0.3vh solid #F3F3F3;
border-left: 0.2vw solid #F3F3F3;
}
div.powerSelection:active {
border-right: 0.2vw solid #F3F3F3;
border-bottom: 0.3vh solid #F3F3F3;
border-top: 0.3vh solid #909090;
border-left: 0.2vw solid #909090;
}
HTML CoXHeroAndVillainBuilder.html (javascript included in page, had to attach it getting too long for the post)
[attachment id=3 msg=4490]
loadPowerSetsForLoop.js (Short Version) attached [attachment id=1 msg=4490]
primaryPowersList.js attached [attachment id=2 msg=4490]
As always any help in this project is appreciated. any final outcome will be posted in source (or source documents) here, by someone. and available to place as a page on any city of heroes website.
I don't own any rights to suckerpunch's nor did I get expreess permission to use their code, but I've changed it so drastically that it's vaguely resembling their code anyways, and it's abandonned. I'm not sure if this can be licensed, the plan is to GNU GPL it = Free to use, modify and distribute.
I'll get back into this one of these days here soon. just catching up on a backlog of stuff.
Janu/29/2025
Today I finished the Secondary Powersets powerlists dictionary. And bug tested all secondaries. there were a few casual mistakes. that's all working now. I might need to make a few more edits later.
Now I need to start a new code document for Pool powers and coding it so you can only have one copy of any given pool. Then ancillaries.
I messed with math recently and we decided on a course of action for maintaining number accuracy. there may be inaccuracies in the first version, or maybe there wont be. I know some methods for float handling so we'll deal with that if we need to.
The power info table has been successfully tabbed using a z-index position: absolute, however it was kinda frustrating and only worked after I closed my browser and reopenned it, so there may be some bugs/ headaches to deal with there. But for now it's working and I'm hessitant to touch it though I do need to touch it as I only made 2 tabs.
this project is getting closer. But the BIG job is yet to come. Power Stat Dictionaries.. those will be a tedious chore of data entry. any help will be appreciated once I define how they'll look, it will take no knowledge of coding to help, simple text and number value entry.